- Published on
Implementing Stacks with NodeJS and Javascript
- Data Structures and Algorithms - A Summary of The Most Used Ones
- Implementing Arrays with NodeJS and Javascript
- Implementing Linked-Lists with NodeJS and Javascript
- Implementing Stacks with NodeJS and Javascript
- Implementing Queues with NodeJS and Javascript
- Implementing Trees with NodeJS and Javascript
- Implementing Graphs with NodeJS and Javascript
- Implementing Hash Tables with NodeJS and Javascript
Let's Talk About Stacks
In this post we are going to implement stacks, so, how they work? what are stacks?
Stacks are one of may data structures that we can use to better handle data in our applications. So basically in simple words stacks are a way to order and process data (collections). Stacks work with a principle of LIFO (last in, first out) by that we mean that the last element included on a stack will be the first one to be removed/processed.
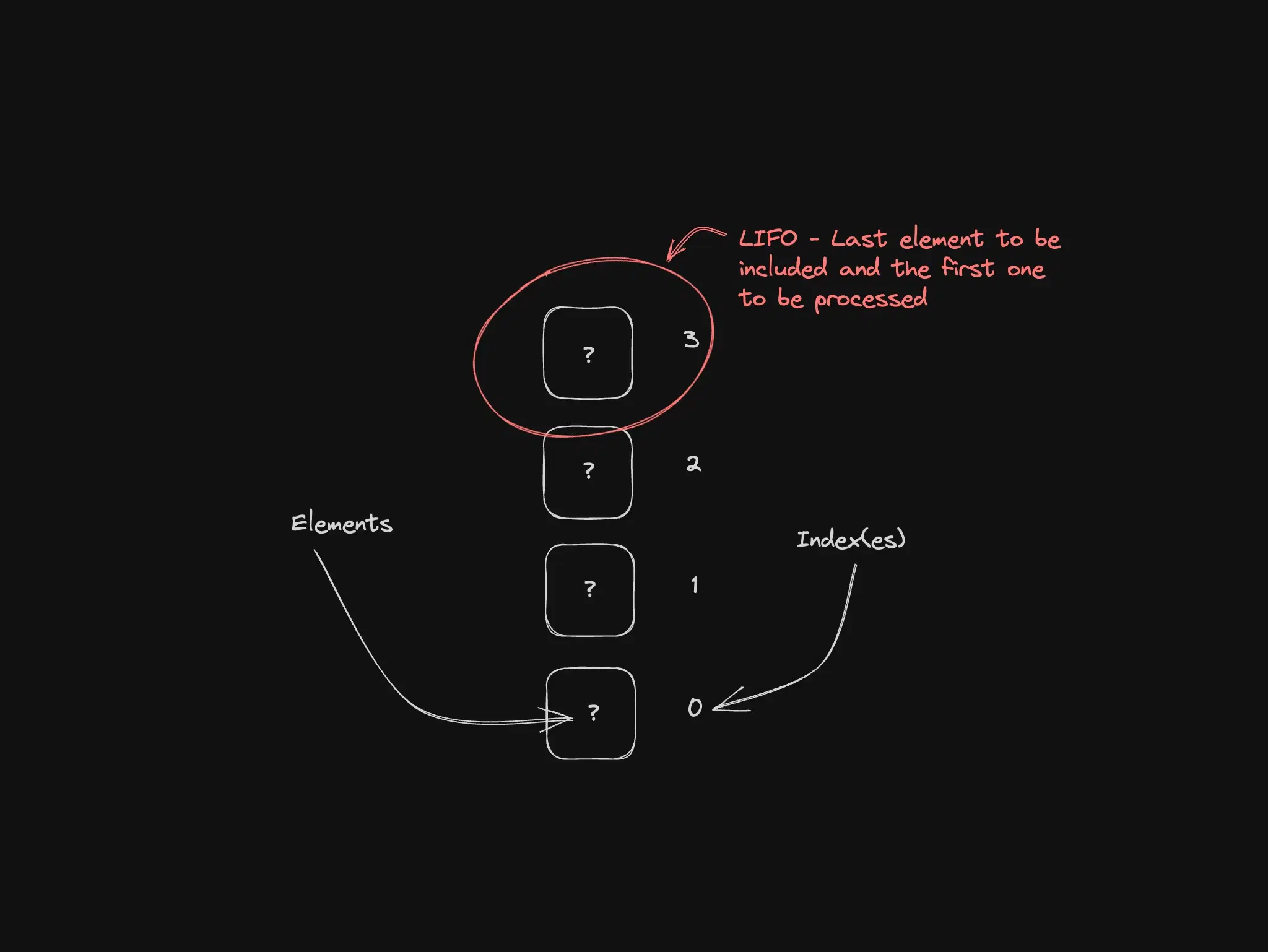
You can easily understand and implement Stacks in NodeJS/Javascript, they can help you to optimize some data collection that you need to reverse, our when you need a specific sequence to operate a collection. You know, when you did that interview that you have been asked to revert an array? Yeah, that's right you could have used stacks to do that.
Well personally I don't like those kind of interviews, but that's a subject for another post...let's dive in on implementing Stacks.
Stacks: How to Implement
One thing you should know before dive in the code, Stacks are an ordered type of list (collection, array - call it what you want) so every operation is based on the top of the list/stack. That's why we call it LIFO.
Implementando Pilhas em JavaScript
When we are talking about vanilla javascript or node without specifics libraries, they don't have a built in stack data structure for us to use. And that's awesome, because we can implement or own, this help us understand how stack works and we can implement it on our own way to suit our needs.
Of course there is a bunch of libraries that have Stacks and other datastructures for you to use, these libs are good when you already have a understanding on how Stacks works and need to use them within your apps without having to implement and do maintanence, but for the sake of our knowlowegde here, let's implement our own, it's not a big deal.
Here is a sample:
class Stack {
constructor() {
this.items = []
}
// Adds an element on top of the Stack
push(element) {
this.items.push(element)
}
// Removes the element on top of the Stack
pop() {
if (this.items.length == 0) {
return 'Empty' // Pilha vazia
}
return this.items.pop()
}
// Returns the element on top of the Stack (without removing it)
peek() {
return this.items[this.items.length - 1]
}
// Checks if the Stack is empty
isEmpty() {
return this.items.length == 0
}
// Logs all the stack elements
printStack() {
for (let i = this.items.length - 1; i >= 0; i--) {
console.log(this.items[i])
}
}
}
What's Next?
Few examples where you can use Stacks:
Managing Functions Execution
Stacks are essential to manage function executions on certain projects. Usually you won't have to handle hard implementations of stacks because usually they are build in in languages or operational systems, but if you want to advance your study that's one way of using it.
Another example you can think of is: javascript stack trace...
Sorting
You know when you fetch a DB history change, so usually you need to see the last change as the first one in the list, it's a concept of stack - the last in is the first out - this is a little abstract because is just an order that you can change, but you can use that to think about solutions for other problems just like the one I mentioned when you need to revert an array.
Parsing Algorithms
Usually parsing algorithms need stacks to keep the order when they are parsing operators and their values. Stacks help in this cases to keep the right others of things.
Tips
- Whenever you implement stacks stay simple, keep only the functions and operations that you need. Think about maintaining the stack don't over-engineer it.
- Use your language built in functions and methods that way you can guarantee more efficiency and rely on stable and already tested functions
- If you are working on big projects with high volume of data you need to start thinking about using a library with a stable implementation of stacks or you need to implement more validations and test to your implementarion if you need specific actions, and maybe even chage to other data structure if it better suit your needs.
In Summary:
You don't have to be specialized on algorithms and data structures but if you understand the concept and implementation of stacks in NodeJS/JavaScript it will help you to create more efficient and well-organized applications. Stacks offer an effective way to manage data in a controlled sequence, making them indispensable in a wide range of programming applications.
Have you ever implemented or used stacks in your NodeJS/JavaScript projects? Share your experiences, challenges or insights in the comments below. Where are the one-liners solving array reversal with stacks in the comments?